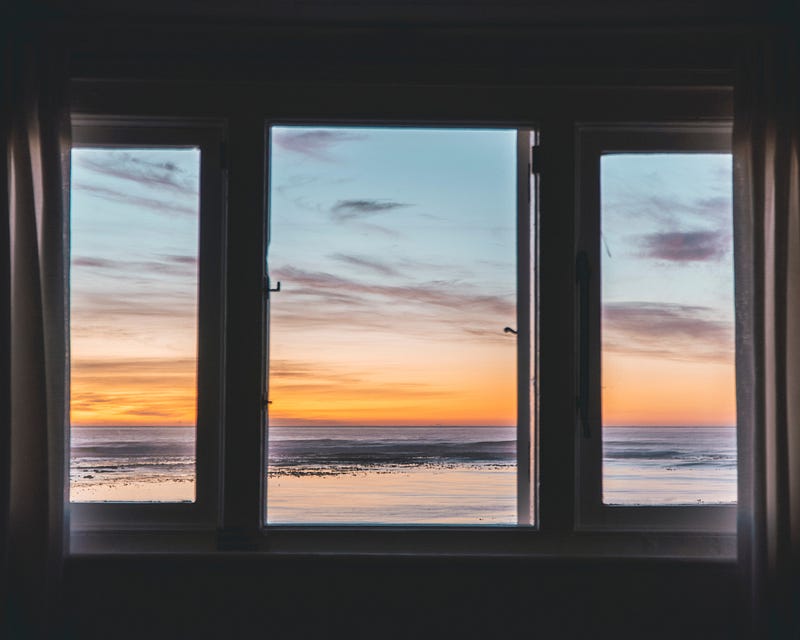
假設今天我們需要獲取網址列的資訊,可以怎麼做?想必大家對 location.href 並不陌生吧?
假設今天我們有一個網址
可以有這些方法取的其他的 URL 資訊
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
|
location.host 👉 "vuejs.org"
location.hostname 👉 "vuejs.org:8080"
location.href 👉 "https://vuejs.org:8080/support-vuejs?tid=123456#One-time-Donations"
location.origin 👉 "https://vuejs.org"
location.pathname 👉 "/support-vuejs/"
location.port 👉 "8080"
location.hash 👉 "#One-time-Donations"
location.protocol 👉 "https:"
locaiton.search 👉 "?tid=222"
|
這邊順便提到 javascript 頁面跳轉、重新整理、導頁的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
|
location.replace
P.S 瀏覽紀錄不會被保存在 history 裡,因此無法按上一頁
locaiton.assign(url) locaiton.href = "url"
P.S 瀏覽紀錄均記錄在 history 裡,可按上一頁
history back 部份版本瀏覽器不支援
history go -1
history.forward()
history.go(0)
location.reload() 其實這個方法可以傳參數 預設為 false 重整時就會從 cache 讀取資源
location.reload(true) 傳入 true 就會強制瀏覽器又去 server 那邊請求資源 等同於 Ctrl +F5
補充一個比較少用的
嵌入的 iframe 獲取父層的網址
parent.window.location
|
關於瀏覽器的歷史紀錄
window.history 物件會紀錄使用者瀏覽網頁的紀錄,但基於隱私,我們無法知道詳細的 URL 是什麼,唯一能獲得的資訊就是 history length ,瀏覽器的瀏覽紀錄筆數,開新頁面的時候 length 為 1。
實作 SPA
如果曾經用過 vue cli 的人,一定對 spa 不陌生,頁面透過 hash 的變化,不重整頁面就可以切換不同的內容,HTML5 裡引用了新的 API,history.pushState 和 history.replaceState,可以拿來實作 SPA (Single Page Application),改變 url 的 hash 而不會 relaod 頁面。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| let state = { pageInfo: "production", };
history.pushState(state, "product", "/product");
history.pushState(state, title, url);
history.replaceState();
|
監聽 hash 的變化
1 2 3 4 5 6 7
| window.addEventListener( "hashchange", (event) => { console.log(event); }, false );
|
popstate
當使用者點擊上一頁或下一頁就會觸發 popstate 事件
1 2 3 4 5
| window.addEventListener("popstate", function (event) { var state = event.state; });
|
history.back()、history.forward()、history.go()是會觸發 popstate 事件,
但是 history.pushState()和 history.replaceState()不會觸發 popstate 事件。